Similar to other languages, a FOR loop repeats a statement a specified number of times. In your programming life, you will see FOR loop is surprisingly powerful, versatile and flexible because it allows
a wide range of variations from its traditional use. A simple form of FOR loop is:
for (initialization, condition, increment) statement
or,
for (initialization, condition, decrement) statement
where,
initialization -- To set a loop control variable to an initial value. The initialization is an assignment statement that sets the initial value of the
loop control variable and acts as the counter that controls the loop.
condition -- An
expression that is tested each time the loop repeats. The condition is
a conditional expression that determines whether or not the loop will repeat.
increment or decrement -- An expression that determines
how the loop control variable is incremented each time the loop repeats. That is, the
increment defines the amount by which the loop control variable will change each
time as the loop is repeated. The FOR loop can proceed in a positive or negative fashion, and it can increment the
loop control variable by any amount. (see Table 1149a)
Note that these three major sections of the loop must be
separated by semicolons. As long as condition is true
(non-zero), the loop keeps running. The FOR loop will continue to execute as long as the conditional
expression tests true. Once the condition becomes false, the loop will exit, and then program
execution will resume on the statement following the FOR block.
For instance, see a basic FOR Loop. In this example, each time the loop repeats, the condition count <= 1967 is
tested. If it is true, the value is output and the count is increased by one. When count
reaches a value greater than 1967, the condition becomes false, and then the loop stops
running. Another example is shown in Table 1149a.
Table 1149a. Examples of FOR loop.
DM script:
number EMNumber
for(EMNumber=1; EMNumber < 8; EMNumber++)
{
number EMsqRoot
EMsqRoot = sqrt(EMNumber)
result (EMsqRoot + "\n\n")
} |
Script output:
1
1.41421
1.73205
2
2.23607
2.44949
2.64575 |
With the square root function
The increment is 1. |
DM script:
number EMi;
for(EMi=100; EMi >= -100; EMi = EMi-40)
result (EMi + "\n") |
Script output:
100
60
20
-20
-60
-100 |
The decrement is -40. |
For repeating a block, a general form is:
for(initialization; expression; increment)
{
statement sequence
}
In professionally written C++ code, you will seldom see a statement like
EMCount = EMCount + 1. Instead, you will see EMCount ++, which also increases its operand by 1 (The ++ is the increment operator). Note that "– –" is the decrement operator,
which decreases its operand by 1.
Table 1149b. FOR statement (loop).
FOR (initialize; condition; action) loop-action |
Loop statement: Create counting loops in a program. The counting process is repeated until a variable reaches a specific limit. (Comparison between with and without FOR command: Example1 and Example2), Example3, Example4, Example5, Example7, Example8. |
FOR (initialize; condition; action)
{
loop-action1
loop-action2
...
} |
Loop statement: Create counting loops in a program. The counting process is repeated until a variable reaches a specific limit. Note the FOR statement should not be used for looping over image pixels in order to obtain optimal speeds of PCs, instead, an image expression should be used. E.g. Example1 (comparison with a script with ExprSize command), Example2,
|
It is important to note that the conditional expression is always tested
at the top of the loop; therefore, the code inside the loop may not be executed at
all if the condition is false to begin with, for example:
number EMi
for(EMi=8; EMi < 4; EMi++)
result (EMi + "\n")
This loop will never execute (meaning that there will be no output from the program) because its control variable (initialization), EMi, is 8 which is greater than 4
when the loop is first entered. This makes the conditional expression, EMi <4, false
from the outset; and thus, not even one iteration of the loop will occur.
In C++ and thus DM script, the pieces of the loop definition need not be there. For instance, if you
want to write a loop that runs until the number 1967 is typed in at the keyboard, the DM script
could look like this (DM script):
number EMMagic
for(EMMagic=0; EMMagic != 1967; )
{
result (EMMagic + "\n")
GetNumber("Enter 1967 to stop printing input numbers and the program", EMMagic, EMMagic)
}
In this program, the increment portion of the for definition is blank. Therefore, each time the
loop repeats, EMMagic is tested to see whether it equals 1967, but no further action takes place.
If 1967 is typed at the keyboard, the loop condition becomes false and the
loop exits. The C++ for loop will not modify the loop control variable in the {} since no increment
portion of the loop is present.
In DM, the initialization section can be moved outside of the loop, and the initialization section in the FOR loop has the variable name only (script code):
number x
x = 1
for(x; x < 10; x++)
result (x + "\n")
The advantages of placing the initialization outside of the loop are:
i) The variable x is initialized by a value entered by the user or a function before the loop is entered. In this situation, the initialization portion of the FOR might be empty when you control the
loop with a function parameter and use the value that the parameter receives when the
function is called as the starting point.
ii) When the initial
value is derived through a process that does not lend itself to containment within the
FOR statement, for instance, when the variable is set by keyboard input.
FOR loops can be used as Time Delay Loops, which are often used in programs and have no other
purpose than to kill time, for instance:
number x
for(x=0; x<1001; x++)
In this case, there is no need to output the result.
One FOR loop can be nested inside of
another. DM based on C++ allows at least 256 levels of nesting. Table 1149c listed examples of nested FOR loops.
Table 1149c. Nested FOR loops.
DM script |
Function of the scripts |
number EMi, EMj;
for(EMi=2; EMi<1000; EMi++)
{
for(EMj=2; EMj <= (EMi/EMj); EMj++)
if(!(EMi%EMj)) break; // if factor found, not prime
if(EMj > (EMi/EMj))
result ("The EMi" + "\t" + EMi + "\t" + "is a prime number\n")
}
Script code |
Print all prime numbers less than 1000 |
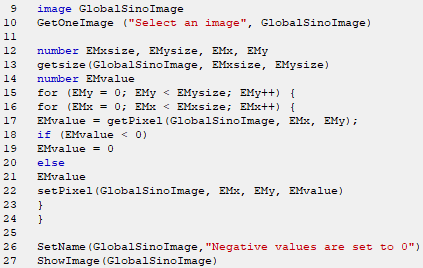
Script code |
Set the pixel value in an image to zero if its value is negative. |
Where to declare variables sometimes needs to be considered. For instance, in the example below, the EMi is declared within the initialization portion of the FOR and is used to control
this loop only. Outside the loop, EMi is unknown (script):
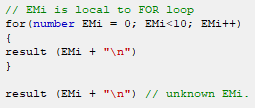
Then, an error message is shown when
it is executed:
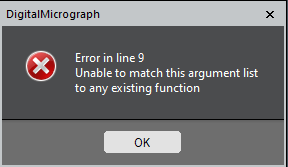
In general, when the loop control variable of a FOR is not needed outside the loop, then
declaring it inside the FOR statement is a good idea because
it localizes the variable to the loop to avoid its accidental misuse elsewhere. You can read more details on variable declaration at page1144.
On the other hand, in many cases, FOR statement can be replaced by WHILE statements (refer to comparison between for and while statements).
|